Last Update: 2022-06-05
This is the main page for carleslibs Open Source Python package.
https://pypi.org/project/carleslibs/
https://gitlab.com/carles.mateo/carleslibs
In order to install use:
pip3 install carleslibs
In case you want to install to a Virtual Environment, activate it first.
For Ubuntu is done like this:
source /home/carles/Desktop/code/my-awesome-project/venv/bin/activate
If you want to upgrade to the latest version:
/home/carles/Desktop/code/my-awesome-project/venv/bin/pip3 install --upgrade carleslibs
Release History
Version 1.0.8
Released on 2022-06-05:
- Fixed a bug in the MenuUtils class, where main title is overwritten with the last subitem from the menu after the first iteration. Inadvertently I was reusing and overwriting a variable.
- Updated the demo application carleslibs_demo.py to use SubProcess Utils to get the Kernel Version.
- Fixed test for OsUtils.get_uptime()
- Added StringUtils.get_substrings_of_n_chars_from_string(self, s_input, i_chars=2)
- Fixed typos in comments.
- Changes to OsUtils.get_total_and_free_space_in_gib() to make decimal cast with round(, 2) and not with String “2f”
You can see a session of Refactor that I streamed by Twitch finding and fixing the Menu bug:
Version 1.0.7
Released on 2022-03-06:
- Modified OsUtils.get_total_and_free_space_in_gib() to return float instead of Integer.
- Added HashUtils class with md5 for unicode Strings.
- Produces the same as md5sum Linux tool.
- Created FileUtils.create_folders() which creates all the subfolders in the path deep.
- Unit Testing:
- Added test_get_inodes_in_use_and_free() to test_osutils.py
- Added two tests more to test_osutils.py
- Added test for version.py
- Tests for HashUtils class.
Version 1.0.6 (minor)
- Added method DatetimeUtils.sleep() and Unit Testing
- Added OsUtils. Mostly focussed on Linux utilites.
- Added some partial Code Coverage.
Version 1.0.5 (minor)
Released on 2022-02-05 with the addition of:
- Created a new method DateTimeUtils.get_unix_epoch_as_int()
- Added a special case for StringUtils.get_percent() to avoid division by 0.
- If StringUtils.get_bytes_per_second() if passed 0 as seconds, this is considered 1 second.
- We avoid a Division by Zero error, and in most of the cases, you want to know the allowed bandwidth, so considered that it took 1 second will be correct.
- Increased Code Coverage for StringUtils to 95%.
Version 1.0.4 (minor)
Was released on 2022-01-23 with the addition of:
- html_escape() has been added to StringUtils class
It safely escapes HTML contents, for displaying on websites without breaking the page.
I’m using it in one of my automation projects for displaying logs and objects in a Flask served web page.
Version 1.0.3
Was released on 2021-12-08 with the addition of:
- carleslibs_demo.py
A demo project using the libraries to easily understand the usage and power. - MenuUtils
A class to build application based menus very quickly. - KeyboardUtils
A class to ask the user for input data from the Keyboard, and validating certain matching criteria.
Version 1.0.1
Was released on 2021-08-11 wit the addition of:
- SubProcessUtils
Allows to execute commands and get Exit Code, StdOut and StdErr.
Version 0.99.2 was released on 2021-08-27 with the addition of:
- StringUtils
Version 0.99.1 was released on 2021-07-12 with:
- FileUtils
- DateTimeUtils
- PythonUtils
Source Code
The source code is available at:
https://gitlab.com/carles.mateo/carleslibs
Open Source License
I released it under the MIT License.
How to use it
In order to use them in your project, just install them with pip, or with PyCharm if you’re using a Virtual Environment there.
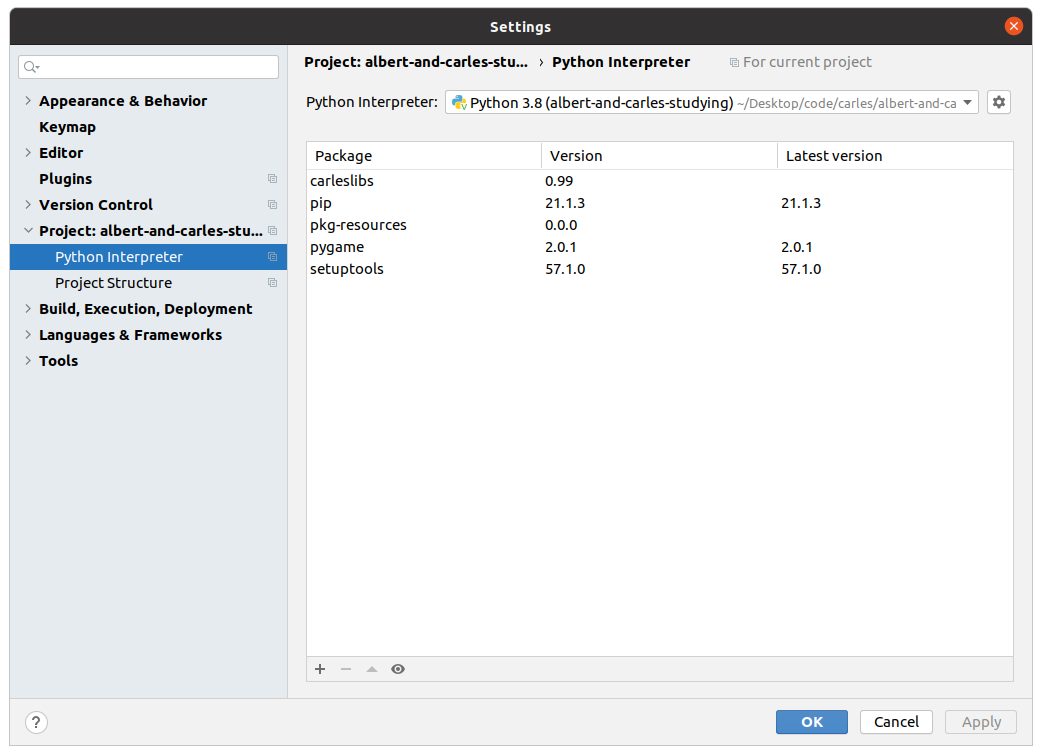
In order to use in your program import the classes you want like this:
from carleslibs.fileutils import FileUtils
from carleslibs.datetimeutils import DateTimeUtils
from carleslibs.pythonutils import PythonUtils
from carleslibs.stringutils import StringUtils
DateTimeUtils class
And use in your program:
from carleslibs.datetimeutils import DateTimeUtils
o_datetime = DateTimeUtils()
print(o_datetime.get_datetime_as_14_string())
print(o_datetime.get_unix_epoch())
print(o_datetime.get_datetime())
print(o_datetime.get_unix_epoch_as_float())
This will output:
20210713203940 1626205180 2021-07-13 20:39:40 1626205180.05574
HashUtils class
from hashutils import HashUtils o_hashutils = HashUtils() b_success, s_md5, s_last_chars = o_hashutils.hash_string_as_hexadecimal("Hello World", 2) # s_md5 will have b10a8db164e0754105b7a99be72e3fe5 # Exactly the same as doing from Linux's bash: # echo -n "Hello World" | md5sum # or # printf "Hello World" | md5sum
PythonUtils class
from carleslibs.pythonutils import PythonUtils
o_python = PythonUtils()
s_version, i_major, i_minor, i_micro, s_releaselevel = o_python.get_python_version()
print("Your Python version is: " + s_version)
if o_python.is_python_2() is True:
print("You're running on the obsolete Python 2")
if o_python.is_python_3() is True:
print("Cool, you're running in Python 3")
In my development environment will output:
Your Python version is: 3.8.10 Cool, you're running in Python 3
FileUtils class
from carleslibs.fileutils import FileUtils
o_fileutils = FileUtils()
s_testfile = "/tmp/" + o_datetime.get_datetime(b_milliseconds=False, b_remove_spaces_and_colons=True, b_remove_dashes=False) + "-test.txt"
if o_fileutils.file_exists(s_testfile) is True:
print("A previous file named " + s_testfile + " exists. Cancelling.")
else:
b_success = o_fileutils.write(s_testfile, "This is a test\n")
if b_success is False:
print("Error writing to: " + s_testfile)
b_success = o_fileutils.append(s_testfile, "This is another test")
if b_success is False:
print("Error appending to: " + s_testfile)
b_success, s_contents = o_fileutils.read_file_as_string(s_testfile)
if b_success is False:
print("Error reading from: " + s_testfile)
else:
print("The file " + s_testfile + " contains:")
print(s_contents)
b_success, a_lines = o_fileutils.read_file_as_lines(s_testfile)
if b_success is False:
print("Error reading from: " + s_testfile)
else:
print("The file " + s_testfile + " contains:")
i_line = 1
for s_line in a_lines:
print("Line " + str(i_line) + " contains: " + s_line)
i_line = i_line + 1
b_success = o_fileutils.delete(s_file=s_testfile)
if b_success is True:
print("File " + s_testfile + " successfully deleted")
else:
print("There was a problem deleting file: " + s_testfile)
This will output:
The file /tmp/2021-07-13-210039-test.txt contains: This is a test This is another test The file /tmp/2021-07-13-210039-test.txt contains: Line 1 contains: This is a test Line 2 contains: This is another test File /tmp/2021-07-13-210039-test.txt successfully deleted
StringUtils class
This class provides several methods for unit conversion, string formatting, getting speeds…
It is used in my Open Source CTOP project.
Rules for writing a Comment