Python has a built-in package named ipaddress
You don’t need to install anything to use it.
This simple code shows how to use it
import ipaddress def check_ip(s_ip_or_net): b_valid = True try: # The IP Addresses are expected to be passed without / even if it's /32 it would fail # If it uses / so, the CIDR notation, check it as a Network, even if it's /32 if "/" in s_ip_or_net: o_net = ipaddress.ip_network(s_ip_or_net) else: o_ip = ipaddress.ip_address(s_ip_or_net) except ValueError: b_valid = False return b_valid if __name__ == "__main__": a_ips = ["127.0.0.2.4", "127.0.0.0", "192.168.0.0", "192.168.0.1", "192.168.0.1 ", "192.168.0. 1", "192.168.0.1/32", "192.168.0.1 /32", "192.168.0.0/32", "192.0.2.0/255.255.255.0", "0.0.0.0/31", "0.0.0.0/32", "0.0.0.0/33", "1.2.3.4", "1.2.3.4/24", "1.2.3.0/24"] for s_ip in a_ips: b_success = check_ip(s_ip) if b_success is True: print(f"The IP Address or Network {s_ip} is valid") else: print(f"The IP Address or Network {s_ip} is not valid")
And the output is like this:
The IP Address or Network 127.0.0.2.4 is not valid The IP Address or Network 127.0.0.0 is valid The IP Address or Network 192.168.0.0 is valid The IP Address or Network 192.168.0.1 is valid The IP Address or Network 192.168.0.1 is not valid The IP Address or Network 192.168.0. 1 is not valid The IP Address or Network 192.168.0.1/32 is valid The IP Address or Network 192.168.0.1 /32 is not valid The IP Address or Network 192.168.0.0/32 is valid The IP Address or Network 192.0.2.0/255.255.255.0 is valid The IP Address or Network 0.0.0.0/31 is valid The IP Address or Network 0.0.0.0/32 is valid The IP Address or Network 0.0.0.0/33 is not valid The IP Address or Network 1.2.3.4 is valid The IP Address or Network 1.2.3.4/24 is not valid The IP Address or Network 1.2.3.0/24 is valid
As you can read in the code comments, ipaddress.ip_address() will not validate an IP Address with the CIDR notation, even if it’s /32.
You should strip the /32 or use ipaddress.ip_network() instead.
As you can see 1.2.3.4/24 is returned as not valid.
You can pass the parameter strict=False and it will be returned as valid.
ipaddress.ip_network(s_ip_or_net, strict=False)
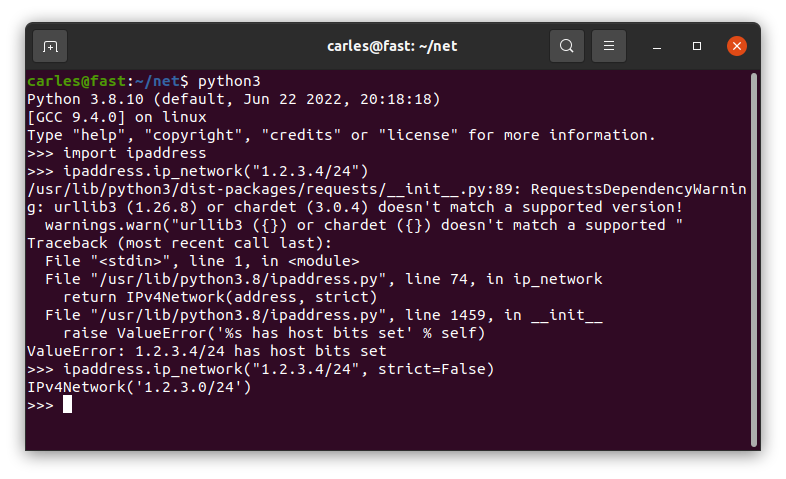