Here you can see this Python code to connect via Telnet and executing a command in a Server:
File: telnet_demo.py
#!/usr/bin/env python3 import telnetlib s_host = "localhost" s_user = "telnet" s_password = "telnet" o_tn = telnetlib.Telnet(s_host) o_tn.read_until(b"login: ") o_tn.write(s_user.encode('ascii') + b"\n") o_tn.read_until(b"Password: ") o_tn.write(s_password.encode('ascii') + b"\n") o_tn.write(b"hostname\n") o_tn.write(b"uname -a\n") o_tn.write(b"ls -hal /\n") o_tn.write(b"exit\n") print(o_tn.read_all().decode('ascii'))
File: Dockerfile
FROM ubuntu:20.04 MAINTAINER Carles Mateo ARG DEBIAN_FRONTEND=noninteractive # This will make sure printing in the Screen when running in detached mode ENV PYTHONUNBUFFERED=1 RUN apt-get update -y && apt install -y sudo telnetd vim systemctl && apt-get clean RUN adduser -gecos --disabled-password --shell /bin/bash telnet RUN echo "telnet:telnet" | chpasswd EXPOSE 23 CMD systemctl start inetd; while [ true ]; do sleep 60; done
You can see that I use chpasswd command to change the password for the user telnet and set it to telnet. That deals with the complexity of setting the encrypted password.
File: build_docker.sh
#!/bin/bash s_DOCKER_IMAGE_NAME="ubuntu_telnet" echo "We will build the Docker Image and name it: ${s_DOCKER_IMAGE_NAME}" echo "After, we will be able to run a Docker Container based on it." printf "Removing old image %s\n" "${s_DOCKER_IMAGE_NAME}" sudo docker rm "${s_DOCKER_IMAGE_NAME}" printf "Creating Docker Image %s\n" "${s_DOCKER_IMAGE_NAME}" sudo docker build -t ${s_DOCKER_IMAGE_NAME} . # If you don't want to use cache this is your line # sudo docker build -t ${s_DOCKER_IMAGE_NAME} . --no-cache i_EXIT_CODE=$? if [ $i_EXIT_CODE -ne 0 ]; then printf "Error. Exit code %s\n" ${i_EXIT_CODE} exit fi echo "Ready to run ${s_DOCKER_IMAGE_NAME} Docker Container" echo "To run in type: sudo docker run -it -p 23:23 --name ${s_DOCKER_IMAGE_NAME} ${s_DOCKER_IMAGE_NAME}"
When you run sudo ./build_docker.sh the image will be built. Then run it with:
sudo docker run -it -p 23:23 --name ubuntu_telnet ubuntu_telnet
If you get an error indicating that the port is in use, then your computer has already a process listening on the port 23, use another.
You will be able to stop the Container by pressing CTRL + C
From another terminal run the Python program:
python3 ./telnet_demo.py
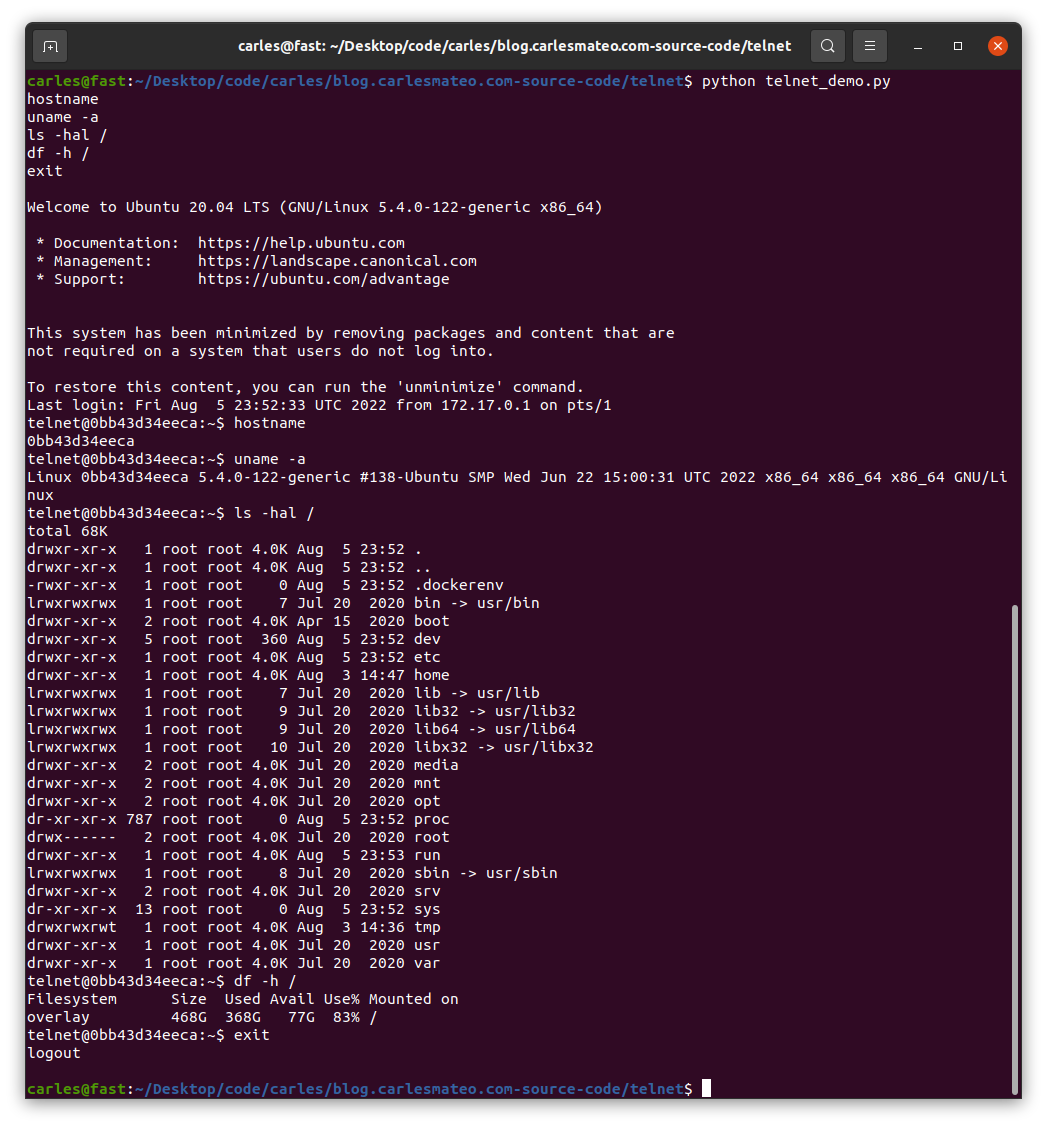